NFC Tag Scanner
Scan data from Near Field Communication (NFC) tags as part of in-store shopping experience, warehouse tracking, digital+physical game, or any similar use case.
Overview
This module enables the reading of NFC tags on iOS and Android. We provide Median JavaScript Bridge commands to initiate tag reading while the app is open. On supported devices we also support background tag reading for apps with universal links. On iOS the default NFC user interface is used. On Android we provide a custom native user interface to provide feedback to the end user.
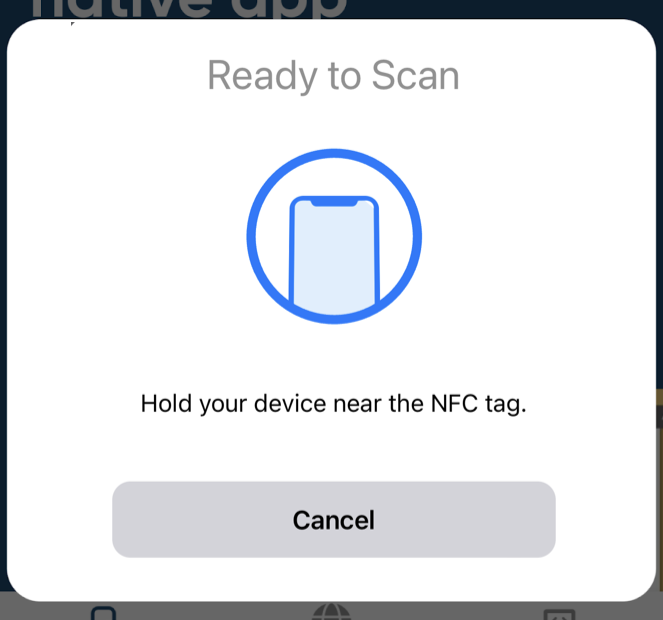
iOS Default UI
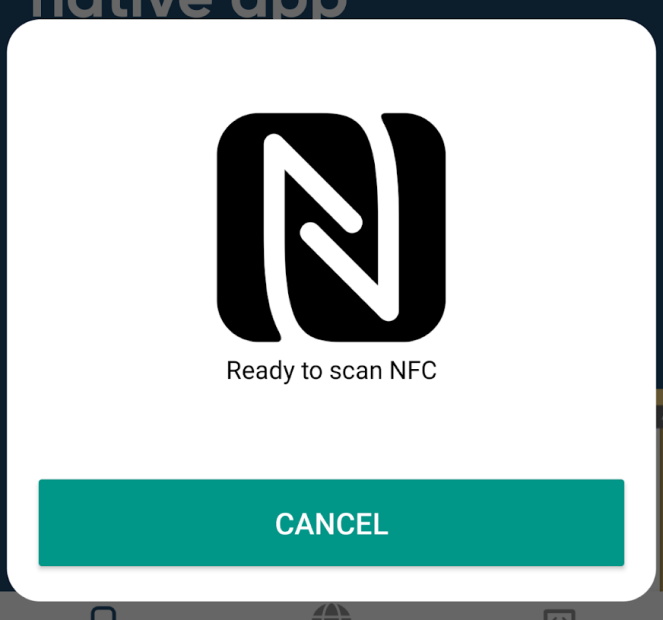
Android Custom UI
iOS Near-field Communications Capability
Prior to building your app add the Near-field Communications Capability within Xcode
Implementation Guide
Check for NFC availability
↔️Median JavaScript Bridge
// Promise method median.nfc.status().then(function (data) { // process the data here. In this example, we are just logging it if (data.available) { // show user NFC-related functionality, e.g. a button to start scanning } }); // Callback method function nfcStatusCallback() is defined on page median.nfc.status({'callback': nfcStatusCallback});
Initiate tag reading
↔️Median JavaScript Bridge
// Promise method median.nfc.readTag({ 'message': STRING, // Android only, shown in the prompt when scanning for tags 'openUrl': BOOLEAN // if set to TRUE and an http or https url is returned the URL will automatically be opened }).then(function (data) { // data.success: BOOLEAN, true if scanning was successful // data.cancel: BOOLEAN, true if user canceled the NFC scan prompt // data.error: STRING, included if error may be “SystemIsBusy”, “SessionTimeout”, “SessionTerminatedUnexpectedly”, or “Error” // data.type: NFC tag type // data.prefix: the NDEF tag prefix, e.g. 'https://' // data.content: the rest of the tag payload after the prefix // data.uri: the concatenation of the prefix and the content }); // Callback method function readTagCallback() is defined on page median.nfc.readTag({ 'callback': readTagCallback, 'message': STRING });
Scan Tag Basic Example
↔️Median JavaScript Bridge
median.nfc.readTag({ 'message': 'Hold your device near the storage bin' }).then(function (data) { if (data && data.error) { console.log('There was an error scanning for NFC tags'); return; } if (data && data.uri) { // do something with data.uri } });
Scan Tag and Open URL Directly
↔️Median JavaScript Bridge
median.nfc.readTag({ 'openUrl': true, 'message': 'Hold your device near the exhibit sign' });
Updated 5 months ago