Background Location
Overview
Allow your app to run in the background while subscribed to device location updates. Use the background process to run data backup activities or send data/location to a remote server.
The plugin can provide your app with powerful functionality, here are two use cases: First, a fitness app can utilize the background location functionality to track distances biked, run, or walked while the app is launched but in the background. Second, the plugin provides the functionality to access the real time location of app users. For instance, an app geared towards delivery drivers can track their locations and you can configure push notifications to send automated dispatch messages to the closest drivers.
By default, Median apps have access to the HTML5 geolocation APIs to retrieve the user’s current location, and to subscribe to location update changes. The HTML5 geolocation APIs have a few limitations:
- When the app is paused / backgrounded, location updates may be paused. Updates will typically be resumed when the app is brought back to the foreground, but the device’s movements when the was paused are lost.
- JavaScript is paused when the app is backgrounded, preventing any processing of new locations.
The Median JavaScript Bridge commands described below allow for JavaScript to receive missing location updates when the app is temporarily backgrounded. It also supports uploading location updates to a server for intelligent background processing, or performing any other task on an ongoing basis. For an example use case, the Dropbox app uses background location services to upload camera photos in the background.
Permissions
When background location is active on iOS, the app may modify the device’s status bar to indicate it is using the device location. This also provides a convenient way for the user to return to the app by tapping the status bar.
Android requires that an ongoing service display a foreground notification. You may customize the title and text for this notification.
The Background Location Native Plugin requires the iOS location permission authorizedAlways
and Android permissions ACCESS_FINE_LOCATION
, ACCESS_COARSE_LOCATION
and ACCESS_BACKGROUND_LOCATION
.
If you only require the background process to run while your app is open on the device you can add an additional parameter to your appConfig.json
as follows: foregroundOnly: true
.
On iOS this configuration uses the location permission authorizedWhenInUse
rather than authorizedAlways
.
On Android the permission ACCESS_BACKGROUND_LOCATION
is not required, only ACCESS_FINE_LOCATION
and ACCESS_COARSE_LOCATION
are requested.
Developer Demo
Display our demo page in your app to test during development https://median.dev/background-location/
Add the Location updates Background Mode capability in Xcode
To enable the background service in your iOS app, open the Capabilities tab in Xcode and expand Background Modes. Enable Location updates as shown below.
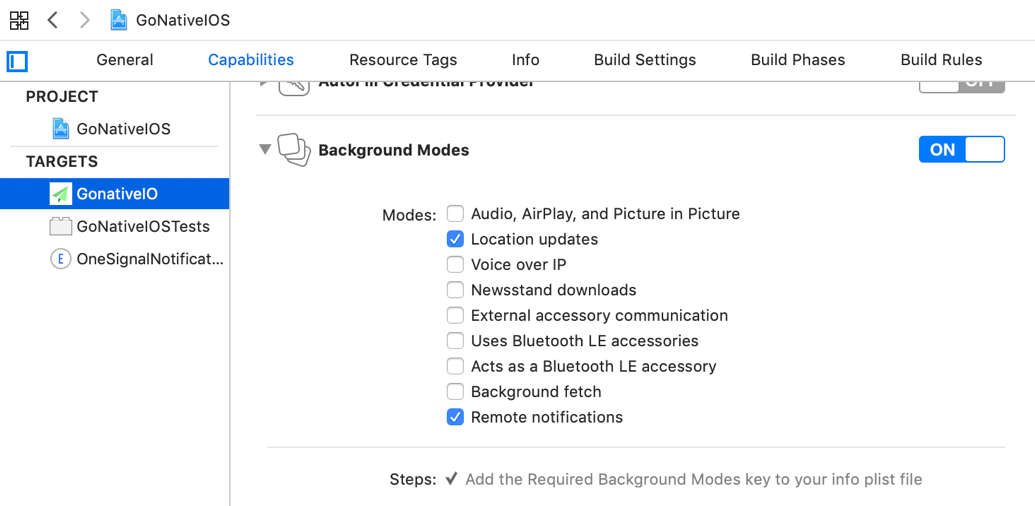
For iOS, add the Location updates under Background Modes in Xcode. Apple changes these options frequently, please let us know if they are different.
Implementation Guide
Once the premium module has been added to your app, you may use the following Median JavaScript Bridge commands to access its functionality.
Data format
Your JavaScript function and/or backend API will be called with an object containing the following fields:
locations
: an array of location objects
A location object has the following fields. Fields are not all guaranteed to be populated and may be null or undefined.
timestamp
: time of this location update, in seconds since Jan 1 1970latitude
: the latitude in degreeslongitude
: the longitude in degreesaltitude
: the altitude in metersfloor
: (iOS only) the floor of the building in which the user is locatedhorizontalAccuracy
: in metersverticalAccuracy
: in metersspeed
: instantaneous speed of the device in meters per secondspeedAccuracy
: (Android only) in meters per secondbearing
: the direction of device movement, in degrees clockwise from north. Has a range of [0.0, 360.0]bearingAccuracy
: (Android only) in degrees
Start background location service
↔️Median JavaScript Bridge
To start background location scanning, run the JavaScript function:
median.backgroundLocation.start(locationRequest);
Replace locationRequest with a JSON object with the following fields:
callback
: (optional ifpostUrl
is provided) the JavaScript function name that you have defined to get the location updates.postUrl
: (optional ifcallback
is provided) a URL to which location updates will be POSTed as a JSON object. Note that the updates will typically not be sent with the same cookies as the user’s webview session, so please embed any necessary identifiers or authentication tokens in this URL.
iOS specific parameters
There are several parameters used on iOS:
iosBackgroundIndicator
: (default false) On iOS, it modifies the status bar when the app is backgrounded to indicate the app is using location. Provides a quick way to return to the app.iosPauseAutomatically
: (default true) On iOS, it allows the automatic pausing of location updates when the location is unlikely to change.iosDistanceFilter
: (in meters, default 0.0) On iOS, it does not update location if the device has moved less than this distance since the last updateiosDesiredAccuracy
: A string to indicate the desired accuracy. Choosing lower accuracy enhances battery life.best
: (default) The best accuracy availablebestForNavigation
: The highest possible accuracy that uses additional sensor data to facilitate navigation apps.tenMeters
: Accurate to within ten meters of the desired target.hundredMeters
: Accurate to within one hundred meters.kilometer
: Accurate to the nearest kilometer.threeKilometers
: Accurate to the nearest three kilometers.
iosActivityType
: A string that helps inform the behavior of automatic location pausing wheniosPauseAutomatically
is enabled.other
: (default) unknown activityautomotiveNavigation
: The location manager is being used specifically during vehicular navigation to track location changes to the automobile.otherNavigation
: The location manager is being used to track movements for other types of vehicular navigation that are not automobile related.fitness
: The location manager is being used to track fitness activities such as walking, running, cycling, and so on.airborne
: The location manager is being used specifically during airborne activities.
Android specific parameters
androidInterval
: The rate in milliseconds the app would prefer to receive updates. Actual update interval may be faster or slower.androidFastestInterval
: The fastest rate in milliseconds the app can receive updates. Actual interval update may be slower.androidPriority
: A string that gives the device a strong hint as to which location sources to use.highAccuracy
: Most likely to use GPSbalanced
: Precision approximately within a city block, or 100 meters. Likely to use WiFi and cell tower positioning.lowPower
: City-level positioning, about 10 kilometers.noPower
: The app will not trigger any location updates, but will receive locations triggered by other apps.
androidSmallestDisplacement
: In meters, default 0. LikeiOSDistanceFilter
, sets the minimum location change since the last update.androidNotificationTitle
: The title for the notification that shows the user the app is using their locationandroidNotificationText
: The text for the notification
Location updates will also be sent to the callback
function and/or postUrl
while the app is in the foreground. When the app is in the background, updates to postUrl
will continue but the JavaScript callback
function will be paused. When the app is resumed, any location updates that the callback
function missed are sent in a large batch.
Sample code
As an example, you may use this JavaScript code to start background locations:
function locationUpdated(data) {
console.log('got location', data);
}
var locationRequest = {
callback: locationUpdated,
postUrl: 'https://example.com/location/user123?token=abcabc',
iosBackgroundIndicator: true,
iosPauseAutomatically: true,
iosDesiredAccuracy: 'bestForNavigation',
iosActivityType: 'automotiveNavigation',
androidPriority: 'highAccuracy',
androidNotificationTitle: 'Navigation',
androidNotificationText: 'Tap to return to app'
};
median.backgroundLocation.start(locationRequest);
Stop background location service
↔️Median JavaScript Bridge
To stop location updates, run the JavaScript function:
median.backgroundLocation.stop();
Updated 2 months ago